Decision control structure in C allows us to perform different set of actions in different situations.
Point : Suppose the if block consists of only one statement,then no need of braces.Braces are placed when block contains multistatements.
Here's a sample program
Here's a sample program
This statement allows us to make a choice from a number of alternatives.Instead of writing a series of if statements we use switch statement.
Here's a sample program
and here's the o/p
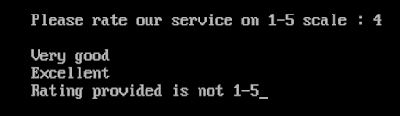
But we expected only Very good
This happened as we did not place a break statement in cases.break statement takes the control out of switch.If we did not place a break in cases,our control will fall through to subsequent cases until break statement is encountered.To avoid this so that only required case is executed,we place break in cases.It is fine if we don't place a break in last case as we don't have any subsequent cases to fall through to.
Here's an updated version of above program
if construct
if(condition)
{
statements //if block
}
If the condition is true if block is executed.Point : Suppose the if block consists of only one statement,then no need of braces.Braces are placed when block contains multistatements.
Here's a sample program
#include<stdio.h>
int main()
{
int pt;
printf("Please rate our service on 1-5 scale");
scanf("%d",&pt);
if(pt==5)
printf("Thankyou for rating us as the best service");
return 0;
}
if else construct
if(condition)
{
statements //if block
}
else
{
statements //else block
}
If the condition is true if block is executed,else else block is executed.Here's a sample program
#include<stdio.h>
int main()
{
int pt;
printf("Please rate our service on 1-5 scale");
scanf("%d",&pt);
if(pt==5)
printf("Thankyou for rating us as the best service");
else
printf("We will surely try to improve");
return 0;
}
if else if else construct
An if statement can be followed by an optional else if statement so that block of code corresponding to 1st condition that evaluates to true is only executed and other statements are skipped.
if(condition1)
{
statements //if block
}
else if (condition2)
{
statements //else if block
}
else if(condition3)
{
statements //else if block
}
else
{
statements //else block
}
Suppose condition2 is true then only the above highlighted code is executed.
Ternary operator
condition ? statement1 : statement2;
If condition is true statement1 is executed else statement2 is executed.
This operator is commonly used in conditional assignment statements.
Instead of writing
if(percent>=35)
status = "pass";
else
status = "fail";
its preferable to write
status = percent>=35 ? "pass" : "fail";
switch construct
This statement allows us to make a choice from a number of alternatives.Instead of writing a series of if statements we use switch statement.
switch(expression)
{
case constant-expression:
statements
break; //optional
case constant-expression:
statements
break; //optional
...
...
default:
statements
}
The expression is evaluated.This value is matched against each constant-expression.When a match is found that case along with subsequent and default cases are executed.When no match is found default case is executed.Here's a sample program
int main()
{
int pt;
printf("\nPlease rate our service on 1-5 scale : ");
scanf("%d",&pt);
switch(pt)
{
case 1:
printf("\nVery poor");
case 2:
printf("\nPoor");
case 3:
printf("\nGood");
case 4:
printf("\nVery good");
case 5:
printf("\nExcellent");
default:
printf("\nRating provided is not 1-5");
}
return 0;
}
and here's the o/p
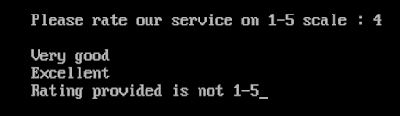
But we expected only Very good
This happened as we did not place a break statement in cases.break statement takes the control out of switch.If we did not place a break in cases,our control will fall through to subsequent cases until break statement is encountered.To avoid this so that only required case is executed,we place break in cases.It is fine if we don't place a break in last case as we don't have any subsequent cases to fall through to.
Here's an updated version of above program
#include<stdio.h>
int main()
{
int pt;
printf("\nPlease rate our service on 1-5 scale ");
scanf("%d",&pt);
switch(pt)
{
case 1:
printf("\nVery poor");
break;
case 2:
printf("\nPoor");
break;
case 3:
printf("\nGood");
break;
case 4:
printf("\nVery good");
break;
case 5:
printf("\nExcellent");
break;
default:
printf("\nRating provided is not 1-5");
}
return 0;
}
and here's the O/P
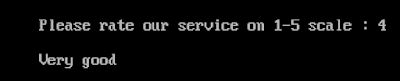
No comments:
Post a Comment